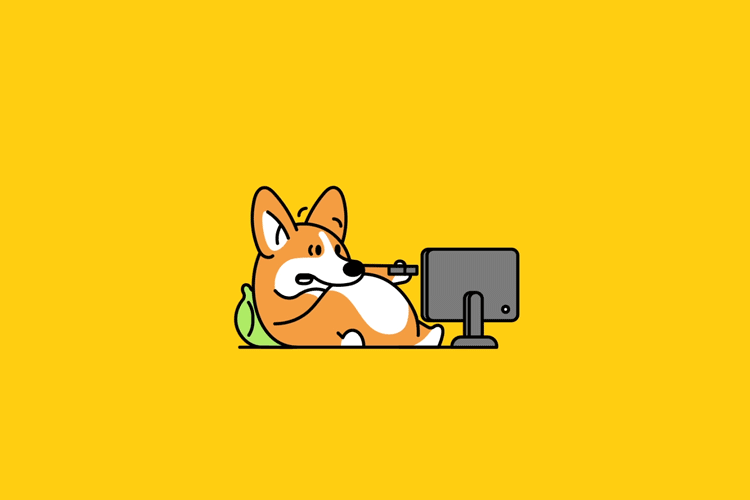
MybatisPlus NULL的没被更新上 解决方案
如果你希望默认开启全部字段的更新,然后只有指定字段为 null
的时候不更新,可以通过配置 MyBatis Plus 的全局策略,并在特定字段上使用 @TableField
注解来覆盖默认策略。
步骤
- 全局配置默认更新策略:将默认策略设置为
IGNORED
,使所有字段在null
时也会更新。 - 针对特定字段覆盖默认策略:在需要的字段上使用
@TableField
注解并设置updateStrategy
为NOT_NULL
。
示例
1. 全局配置
首先,在 MyBatis Plus 的配置文件中配置全局更新策略。假设使用的是 application.yml
:
mybatis-plus:
global-config:
db-config:
field-strategy: ignored
这将设置所有字段在更新时,即使值为 null
也会被更新。
2. 实体类配置
在特定字段上使用 @TableField
注解并设置 updateStrategy
为 NOT_NULL
,使这些字段在 null
时不会被更新。
import com.baomidou.mybatisplus.annotation.TableField;
import com.baomidou.mybatisplus.annotation.FieldStrategy;
public class Acorn {
private Long id;
@TableField(value = "create_time", updateStrategy = FieldStrategy.NOT_NULL)
private LocalDateTime createTime;
@TableField(value = "sort", updateStrategy = FieldStrategy.NOT_NULL)
private Double sort;
// 其他字段和 getter/setter 方法
}
示例测试方法
@Test
public void contextLoads3() {
Acorn acorn = new Acorn();
acorn.setId(1L);
acorn.setCreateTime(null); // 这不会更新
acorn.setSort(null); // 这不会更新
acornService.updateById(acorn);
}
生成的 SQL
这样配置后,生成的 SQL 将不会包含 create_time
和 sort
字段的 null
值更新:
UPDATE tt_acorn SET update_time = '2024-07-20T17:03:20.994+0800' WHERE id = 1 AND is_deleted = 0;
结论
通过全局配置将字段更新策略设置为 IGNORED
,然后在特定字段上使用 @TableField
注解并设置 updateStrategy
为 NOT_NULL
,你可以实现默认开启所有字段的更新,而指定字段在 null
时不更新。这样可以灵活控制各个字段的更新策略。
为了实现默认开启全部字段的更新(包括 null
值),但只有个别字段在 null
时不更新,可以结合全局配置和字段级别的注解来实现。
步骤
- 全局配置默认更新策略为
**IGNORED**
:使所有字段在null
时也会更新。 - 针对特定字段覆盖默认策略为
**NOT_NULL**
:在需要的字段上使用@TableField
注解并设置updateStrategy
为NOT_NULL
。
示例
1. 全局配置
在 MyBatis Plus 的配置文件(例如 application.yml
)中配置全局更新策略为 IGNORED
。
yaml
复制代码
mybatis-plus:
global-config:
db-config:
field-strategy: ignored
这将设置所有字段在更新时,即使值为 null
也会被更新。
2. 实体类配置
在特定字段上使用 @TableField
注解并设置 updateStrategy
为 NOT_NULL
,使这些字段在 null
时不会被更新。
java
复制代码
import com.baomidou.mybatisplus.annotation.TableField;
import com.baomidou.mybatisplus.annotation.FieldStrategy;
public class Acorn {
private Long id;
@TableField(value = "create_time", updateStrategy = FieldStrategy.NOT_NULL)
private LocalDateTime createTime;
@TableField(value = "sort", updateStrategy = FieldStrategy.NOT_NULL)
private Double sort;
private String name;
private Integer age;
// 其他字段和 getter/setter 方法
}
在这个例子中,createTime
和 sort
字段在 null
时不会被更新,但 name
和 age
等其他字段在 null
时会被更新。
示例测试方法
java
复制代码
@Test
public void contextLoads3() {
Acorn acorn = new Acorn();
acorn.setId(1L);
acorn.setCreateTime(null); // 这不会更新
acorn.setSort(null); // 这不会更新
acorn.setName(null); // 这会更新
acorn.setAge(null); // 这会更新
acornService.updateById(acorn);
}
生成的 SQL
这样配置后,生成的 SQL 将包含 name
和 age
字段的 null
值更新,但不会包含 create_time
和 sort
字段的 null
值更新:
sql
复制代码
UPDATE tt_acorn SET name = NULL, age = NULL, update_time = '2024-07-20T17:03:20.994+0800' WHERE id = 1 AND is_deleted = 0;
结论
通过全局配置将字段更新策略设置为 IGNORED
,并在特定字段上使用 @TableField
注解并设置 updateStrategy
为 NOT_NULL
,你可以实现默认开启所有字段的更新,而指定字段在 null
时不更新。这种方法可以灵活地控制各个字段的更新策略,从而满足你的需求。
4o